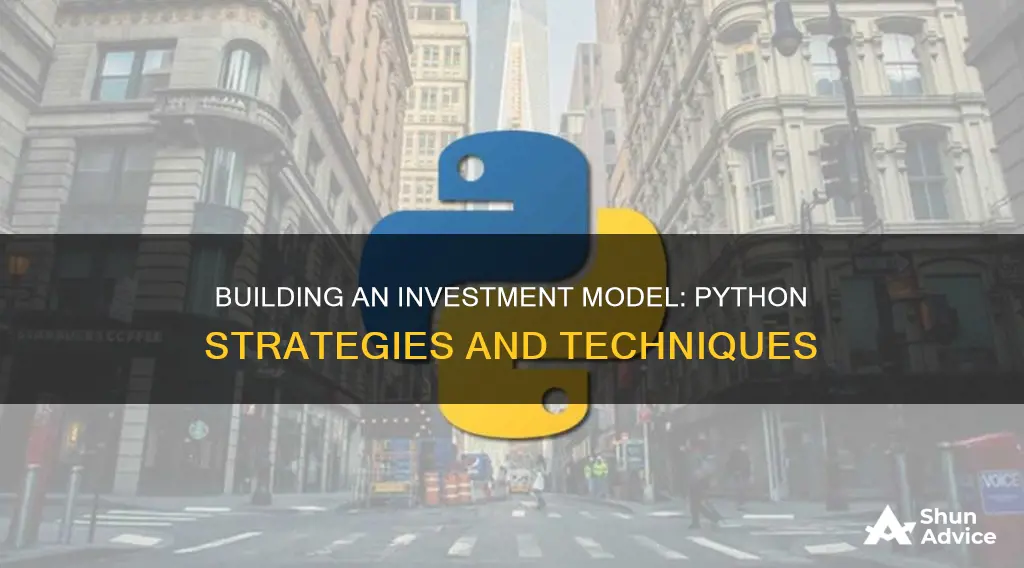
Python is a versatile programming language that can be used to build investment models. Its ability to handle large volumes of financial data and integrate with Excel makes it a popular choice for financial modelling. Python's rich collection of built-in data types and machine learning capabilities allow investors to identify patterns in financial time series data and make informed investment decisions. With Python, investors can screen stocks based on financial ratios, calculate discounted cash flows, and construct investment portfolios that maximize returns while minimizing risk.
Characteristics | Values |
---|---|
Purpose | To build an investment model to find attractive stocks, calculate future cash flows, or construct a portfolio |
Data Sources | Financial ratios, company financials, market data, historical data |
Tools/Techniques | Python programming language, Pandas, Scikit-Learn, Machine Learning, DCF model, Amortization table |
Benefits | Automate tasks, handle large volumes of financial data, uncover patterns, improve investment decisions |
What You'll Learn
Using Python to calculate discounted cash flow
Python has become one of the most popular programming languages in finance due to its ability to handle large volumes of financial data and its compatibility with spreadsheets. One of the most important applications of Python in finance is the calculation of discounted cash flow (DCF).
DCF analysis is based on the concept of the time value of money, which is the idea that money in the present is worth more than the same amount in the future. This is because investing money now will generate interest, whereas holding onto it will not. Therefore, investors are incentivized to invest their money now rather than later.
DCF models are used to predict future cash flows after an investment has been made. These models are used by investors, investment bankers, research analysts, and companies to make informed business decisions. For example, investors can use DCF models to identify new investment opportunities, while investment bankers can use them to value assets.
To calculate DCF in Python, you can use the numpy.npv(rate, values) function to calculate the net present value (NPV) of a series of cash flows. You can create these cash flows by using a numpy.array([...]]) of values. The NPV represents the present value of the expected future cash flows, discounted at a certain rate.
Python
Import numpy as np
Define the cash flows
Cash_flows = np.array([-100, 30, 40, 50, 60])
Calculate the NPV at different discount rates
Discount_rates = [0.03, 0.05, 0.07]
Investment_1 = np.npv(discount_rates [0], cash_flows)
Investment_2 = np.npv(discount_rates [1], cash_flows)
Investment_3 = np.npv(discount_rates [2], cash_flows)
Print(investment_1, investment_2, investment_3)
In this example, `cash_flows` is a numpy array representing the series of cash flows. The `discount_rates` list contains three different discount rates (3%, 5%, and 7%). By applying the `np.npv` function with each discount rate, we can calculate the NPV for each scenario.
It's important to note that this is a simplified example, and in practice, you would use more complex data and models. Additionally, there are other Python libraries and tools, such as Pandas, Koalas, and PySpark, that can be used in conjunction with Python to build more sophisticated investment models and calculations.
Cashing Out Your Fidelity Investments: A Step-by-Step Guide
You may want to see also
Building a model based on financial ratios
Step 1: Data Collection and Preparation
Firstly, you need to collect financial data for the stocks you want to analyse. In this example, we will focus on technology stocks listed on the Nasdaq exchange. You can use Python libraries like yfinance or financial APIs to extract financial data and ratios for these companies. Ensure that the companies are comparable by selecting those in the same sector and with similar market capitalisation (e.g., over $10 billion).
Step 2: Selecting Financial Ratios
Choose a set of financial ratios that you believe are important indicators of stock attractiveness. For example, you may select the following ratios: Return on Equity (ROE), Return on Assets (ROA), Debt Ratio, Interest Coverage, Dividend Payout Ratio, Price-to-Book Ratio (PB), Price-to-Sales Ratio (PS), and Price-Earnings Ratio (PE).
Step 3: Data Analysis and Modelling
Now, you can start analysing the financial ratios and building your investment model. Here are the key steps:
- Create a Pandas DataFrame: Convert the collected financial ratio data into a Pandas DataFrame for easier manipulation and analysis.
- Normalise the Data: Normalise the financial ratios to enable comparison across different ratios. This can be done by dividing each ratio by its mean.
- Define Ranking Criteria: Assign weights to each financial ratio based on their importance in your model. For example, you may decide that a higher ROE is more attractive, so you would assign a positive weight to ROE. Conversely, a higher PB ratio may indicate that a stock is overvalued, so you would assign a negative weight to PB.
- Calculate Ranking Scores: Multiply each normalised financial ratio by its respective weight and sum them up to create a ranking score for each stock.
- Rank the Stocks: Sort the stocks based on their ranking scores to identify the most attractive investment opportunities.
Step 4: Model Evaluation and Refinement
Finally, evaluate the performance of your model by comparing the predicted stock attractiveness with historical data. You can use techniques like backtesting to assess how well your model would have performed in the past. If needed, refine your model by adjusting the weights or selecting different financial ratios.
By following these steps, you can build a powerful investment model based on financial ratios using Python. Remember to continuously update your model with the latest financial data and stay informed about any changes in the companies' fundamentals.
Cash Investments: Impacting Stockholders Equity Positively
You may want to see also
Using Python to create an investment portfolio
Python is a versatile programming language that can be used to build robust investment models and portfolios. Here's a detailed guide on using Python to create an investment portfolio:
Understanding Investment Portfolios
An investment portfolio is a collection of investments held by an individual or an institution. These investments can include stocks, bonds, mutual funds, real estate, commodities, and more. The primary goal of an investment portfolio is to generate returns and grow capital while managing risk.
Advantages of Using Python for Portfolio Creation
Python is an ideal programming language for creating an investment portfolio due to its versatility, extensive libraries, and data analysis capabilities. Here are some key advantages:
- Versatility: Python offers a wide range of libraries and frameworks, such as Pandas, Scikit-Learn, and Django, that can be leveraged for financial analysis, machine learning, and web-based applications.
- Data Analysis: Python provides powerful tools for data analysis, visualization, and manipulation. Libraries like Pandas and NumPy enable efficient handling of financial data, making it easier to identify patterns and make informed investment decisions.
- Machine Learning: Python is a popular choice for machine learning applications, including in finance. With libraries like Scikit-Learn and Statsmodels, you can build models to identify potential return drivers, predict performance indicators, and optimize your investment strategies.
- Automation: Python's automation capabilities can streamline repetitive tasks associated with portfolio management, such as data collection, analysis, and reporting.
Steps to Create an Investment Portfolio with Python
Now, let's outline the steps to create an investment portfolio using Python:
- Define Your Investment Objectives: Start by clearly defining your investment goals and risk tolerance. Are you seeking capital appreciation, income generation, or a balance of both? Do you prefer a more conservative or aggressive investment strategy? These factors will guide your portfolio construction.
- Data Collection and Analysis: Gather relevant financial data for the assets you're considering for your portfolio. This may include stock prices, financial statements, market trends, economic indicators, etc. Utilize Python libraries like Pandas and NumPy to efficiently collect, clean, and analyze this data.
- Risk Assessment: Risk management is a critical aspect of portfolio construction. Use Python to analyze the historical performance and volatility of the assets under consideration. Calculate and compare key risk metrics such as standard deviation, beta, and value at risk (VaR).
- Asset Allocation: Based on your investment objectives and risk assessment, determine the appropriate asset allocation for your portfolio. Decide on the weightings of different asset classes (e.g., stocks, bonds, real estate) and specific investments within each class.
- Portfolio Optimization: Utilize Python's optimization techniques to construct an optimized portfolio that aligns with your objectives and risk tolerance. Consider using Modern Portfolio Theory (MPT) as a framework to balance risk and return. MPT helps identify efficient portfolios that maximize expected returns for a given level of risk.
- Backtesting and Simulation: Before deploying your portfolio, use Python to backtest its performance over historical data. This will provide insights into how the portfolio would have fared during different market conditions. Additionally, consider using Monte Carlo simulations to generate a range of possible outcomes and assess the likelihood of achieving your investment goals.
- Monitoring and Rebalancing: Implement a monitoring system using Python to regularly track the performance of your portfolio and its individual investments. Set up alerts or triggers to notify you when adjustments are needed. Periodically rebalance your portfolio to maintain your desired asset allocation and risk profile.
Example: Python Code Snippet for Portfolio Construction
Here's a simplified example of how you might use Python to construct an investment portfolio:
Python
Import pandas as pd
Import numpy as np
Define your investment objectives and risk tolerance
Investment_goal = "Capital Appreciation"
Risk_tolerance = "Moderate"
Collect and analyze financial data
Stock_data = pd.read_csv("stock_data.csv") # Replace with your data source
Stock_data = stock_data.dropna()
Calculate and assess risk metrics
Stock_data ["Volatility"] = np.std(stock_data ["Returns"], ddof=1) * np.sqrt(252)
Determine asset allocation based on objectives and risk
If investment_goal == "Capital Appreciation":
If risk_tolerance == "Moderate":
Stock_allocation = 0.7
Bond_allocation = 0.3
Else:
# Adjust allocations for higher or lower risk tolerance
Pass
Else:
# Adjust allocations for different investment goals
Pass
Construct the portfolio
Portfolio = {"Stocks": stock_allocation, "Bonds": bond_allocation}
Backtest and simulate portfolio performance
Cash Flows: Investment Decision-Making's North Star
You may want to see also
Forecasting cash flow
Discounted Cash Flow (DCF) Models
Discounted cash flow (DCF) models are a popular tool for forecasting cash flows. DCF models predict future cash flows after an investment is made by discounting expected future cash flows to their present value. This analysis is based on the concept of the time value of money, which recognises that money to be received in the future is worth less than money received today.
Python can be used to build a DCF model to calculate key metrics such as Net Present Value (NPV), Internal Rate of Return (IRR), Payback Period, and Multiple Invested Capital (MOIC). These metrics provide valuable insights for investors, investment bankers, and companies when making investment decisions, valuing assets, or allocating capital.
Monte Carlo Simulations
Python also enables more advanced cash flow forecasting techniques, such as Monte Carlo simulations. Monte Carlo simulations use random sampling to generate a range of potential outcomes for uncertain variables. This allows investors to assess the probability of different cash flow scenarios and make more informed decisions.
For example, you can use Python to build a Monte Carlo simulation that replaces point estimates in a DCF model with probability distributions. By running multiple iterations, you can visualise the distribution of potential outcomes and identify the most likely cash flow projections.
Data Analysis and Visualisation
Python's extensive data analysis and visualisation libraries, such as Pandas, NumPy, and Matplotlib, further enhance its capabilities for cash flow forecasting. These libraries enable efficient data manipulation and statistical analysis, and the creation of charts and plots to communicate results effectively.
Additionally, Python's integration with Excel allows for real-time data exchange between spreadsheets and Python code. This enables users to leverage the strengths of both tools, combining Excel's user-friendliness with Python's advanced analytical capabilities.
Advantages of Python for Cash Flow Forecasting
Python is particularly well-suited for cash flow forecasting due to its high-level programming language, ease of use, and flexibility. It abstracts away complex technical details, making it accessible to users without a strong technical background. Its concise and readable syntax, as well as its robust performance, make it a popular choice for financial modelling and analysis.
Python's ability to automate repetitive tasks, pull data from various sources, and perform complex simulations efficiently makes it a powerful tool for forecasting cash flows and building comprehensive investment models.
Seniors: Best Places to Invest Your Cash Wisely
You may want to see also
Using Python to calculate loan payoff schedules
Python is a popular programming language for financial modelling, with its rich collection of built-in data types and ability to modify and analyse excel spreadsheets. It is particularly useful for handling large volumes of financial data.
One example of a financial model that can be built in Python is a loan calculator. This can be done using the Tkinter GUI library, which is the most commonly used method for developing a GUI in Python.
Step 1: Create the main window
First, import the Tkinter module and create a window with a title, such as "Loan Calculator".
Step 2: Add input boxes and labels
Use the Label function to create display boxes for the user to input information. These can be laid out in a table-like structure using the grid method. The labels could include "Annual Interest Rate", "Number of Years", "Loan Amount", "Monthly Payment", and "Total Payment".
Step 3: Create input variables
Create variables to store the user's input, such as self.annualInterestRateVar, self.numberOfYearsVar, self.loanAmountVar, and so on.
Step 4: Create a "Compute Payment" button
This button will call the computePayment function, which will calculate the total payment and monthly payment.
Step 5: Create the `computePayment` function
This function will take the user's input and perform calculations to determine the total payment and monthly payment. The formula for the monthly payment is:
> `loan * interestRate * (1 + interestRate) * numberOfPayments / ((1 + interestRate) * numberOfPayments - 1)`
Step 6: Create the `getMonthlyPayment` function
This function will compute the monthly payment using the formula:
> `monthlyPayment = loanAmount * monthlyInterestRate / (1 - 1 / (1 + monthlyInterestRate) (numberOfYears * 12))`
Step 7: Run the application
Use the mainloop function to run the application.
This loan calculator will allow the user to input their loan amount, annual interest rate, and number of years, and it will calculate the monthly payment and total payment based on those inputs.
Another example of a financial model that can be built in Python is a discounted cash flow (DCF) model. This model predicts future cash flows after an investment has been made and is used by investors, investment bankers, research analysts, and companies to make business decisions. The DCF analysis works on the concept of the time value of money, where an investment made today is expected to generate more money in the future.
Amazon's Investment Strategies: Where is the Money Going?
You may want to see also
Frequently asked questions
Financial modelling in Python involves using the Python programming language to build a financial model. Python is a high-level programming language with a rich collection of built-in data types. It is often used for modification and analysis of Excel spreadsheets and automation of certain repetitive tasks.
Python has become one of the most popular programming languages in finance due to its ability to handle large volumes of financial data. It is also useful for data wrangling and can be superior to manual processes in Excel. Python's broad ecosystem of libraries, such as Pandas, Scikit-Learn, and Django, also makes it well-suited for developing customised machine learning tools.
Examples of financial models that can be built using Python include investment models based on financial ratios, discounted cash flow (DCF) models, and mortgage amortization schedules.
When building an investment model using Python, it is important to consider the level of risk and return that aligns with the investor's goals. Modern Portfolio Theory, founded by Harry Markowitz, provides a framework for maximising return for a given level of risk or minimising risk for a given level of return. Additionally, machine learning techniques can be leveraged to uncover patterns in financial time series data and make more informed investment decisions.